6.5. pyopus.design.cbd
— Sizing across corners¶
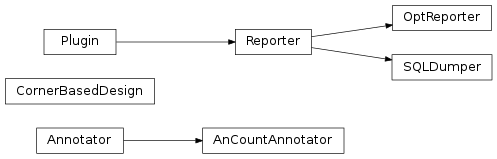
Corners-based design (PyOPUS subsystem name: CBD)
Finds the circuit parameters for which the performances satisfy the requirements across all specified corners.
-
pyopus.design.cbd.
generateCorners
(specs, heads, prefix='c', enumStart=1)¶ Generates corners reflecting all combinations of parameter ranges given by paramSpec and device models given by modelSpec.
Returns a tuple (corners, cornerNames) where corners is a dictionary holding corners with corner name for key and cornerNames is a list of corner names in the order in which the corners were generated.
specs is a list of model/parameter specifications. Every specifications is a tuple/list holding 3 or 4 elements. The elements are
- ‘model’ or ‘param’ specifying if this entry is a list of modules describing a model or a list of parameter values.
- A name of a model or parameter.
- A list of module names or parameter values.
- A list of aliases used for constructing the corner names.
None
indicates the module name/parameter value has no alias. If this list is omitted no aliases are defined for this specification.
heads is a list of head names to which the generated corners apply.
prefix is the strin prefix for the names of the generated corners.
enumStart is the number of the first generated corner. If this value is set to
None
the corners are not enumerated. Note that this results in duplicate corner names if some parameter value/module lacks an alias. Duplicate corner names must be detected by the user by inspecting the cornerNames list.A corner name is constructed as <prefix><number>_<alias>_<alias>_... with first corner numbered enumStart.
Example:
corners=generateCorners( specs=[ ( 'model', 'mos', ['tm', 'wp', 'ws', 'wo', 'wz'], ['tm', 'wp', 'ws', 'wo', 'wz'] ), ( 'model', 'bipolar', ['weak', 'strong'], ['w', 's'] ), ( 'param', 'vdd', [1.6, 1.8, 2.0], ['vl', None, 'vh'] ), ( 'param', 'temperature', [0.0, 25, 100.0], ['tl', None, 'th'] ) ], heads=['opus'], prefix='cor', enumStart=1 ) # Corners is a dictionary with cc<number> as key and # corner description as value. The above statement # results in 3*3*5*2=90 corners named c1..-c90.. representing # the cartesian product of possible mos and bipolar transistor # models, and vdd and temperature values. # Every corner is suffixed by _tm, _wp, _ws, _wo, _wz depending # on the mos model, _w, _s depending on the bipolar model, # _vl or _vh depending on the vdd value, and _tl, _th depending # on the temperature value. # These corners can be passed directly to a PerformanceEvaluator # object.
-
class
pyopus.design.cbd.
CornerBasedDesign
(paramSpec, heads, analyses, measures, corners=None, fixedParams={}, variables={}, norms=None, failurePenalty=1000000.0, tradeoffs=0.0, exclude=None, stopWhenAllSatisfied=None, initial=None, method='local', fullEvaluation=False, forwardSolution=True, incrementalCorners=True, maxiter=None, stepTol=0.001, stepScaling=4.0, evaluatorOptions={}, aggregatorOptions={}, optimizerOptions={}, paramOrder=None, measureOrder=None, cornerOrder=None, debug=0, cleanupAfterJob=True, spawnerLevel=1, sqldb=None, saveAll=False, saveWaveforms='never', waveformsFolder='waveforms.pck', parentId=None)¶ paramSpec is the design parameter specification dictionary with
lo
andhi
members specifying the lower and the upper bounds.See
PerformanceEvaluator
for details on heads, analyses, measures, corners, and variables.Fixed parameters are given by fixedParams - a dictionary with parameter name for key and parameter value for value. Alternatively the value can be a dictionary in which case the
init
member specifies the parameter value.If fixedParams is a list the members of this list must be dictionaries describing parameters. The set of fixed parameters is obtained by merging the information from these dictionaries.
The performance constraints are specified as the
lower
and theupper
member of the measurement description disctionary.norms is a dictionary specifying the norms for the performance measures. Every norm is by default equal to the largest absolute specification (lower, upper). If this results in zero 1.0 is used as the norm. norms overrides this default. Norms are used in the construction of the
Aggregator
object.failurePenalty is the penalty assigned to a failed performance measure. It is used in the construction of the
Aggregator
object used by the optimization algorithm.tradeoffs can be a number or a dictionary. It defines the values of the tradeoff weights for the
Aggregator
object used by the optimization algorithm. By default all tradeoff weights are 0.0. A number specifies the same tradeoff weight for all performance measures. To specify tradeoff weights for individual performance measures, use a dictionay. If a performance measure is not specified in the dictionary 0.0 is used.If exclude is given it contains the list of names of measures that will not produce contributions to the aggregate cost function. These measures will still be compared with their respective goals and included in the aggregate cost function definition.
Setting stopWhenAllSatisfied to
True
makes the optimizer stop as soon as all design requirements (i.e. performance constraints) are satisfied. Setting it toFalse
makes the algorithm stop when its stopping condition is satisfied. When set toNone
the behavior depends on the value of the tradeoffs parameter. If it is set to 0, the optimizer stops when all design requirements are satisfied. Otherwise the behavior is the same as forFalse
.initial is the dictionary specifying the initial point for the optimizer. If not given, the initial point is equal to the mean of the lower and the upper bound.
method can be
local
,global
, or any other supported optimization algorith (currently QPMADS, BoxComplex, ParallelSADE, and DifferentialEvolution) and specifies the type of optimization algorithm to use. Setting it to'none'
skips optimization and only performs an initial circuit evaluation. Currently the local method is QPMADS and the global method is ParallelSADE.If fullEvaluation is set to
`True
the verification step performs all analyses in all corners. See the fullEvaluation option of thePerformanceEvaluator
class.If forwardSolution is
True
the solution of previous pass is used as the initial point for the next pass.If incrementalCorners is
False
the design is optimized for the complete set of corners in a single optimization run. When set toTrue
the set of relevant corners is built gradually with multiple optimization runs (which is much faster for designs with many corners).maxiter is the maximal number of circuit optimizations per optimization run.
stepTol is the step size at which the local optimizer is stopped.
The difference between the upper and the lower bound an a parameter is divided by stepScaling to produce the initial step length for the local optimizer.
evaluatorOptions specifies the option overrides for the
PerformanceEvaluator
object.aggregatorOptions specifies the option overrides for the
Aggregator
object.optimizerOptions specifies the option overrides for the optimizer.
paramOrder is a list of parameter names in which the parameters will be ordered when printed in debug messages.
measureOrder is a list of measure names in which the measures will be ordered when printed in debug messages.
Setting cleanupAfterJob to
False
leaves the intermediate files on the disk after a run is finished.Setting spawnerLevel to a value not greater than 1 distributes the full corner evaluation across available computing nodes. It is also passed to the optimization algorithm, if the algorithm supports this parameter.
sqldb is the SQLiteDatabase object for writing the results.
If saveAll is set to
True
results of every optimization iteration are stored in the results database (if sqldb is given) and printed in the debug output.When sqldb is specified the results generated by the simulator can be saved for later inspection. Setting saveWaveforms to
verification
saves them as pickled result files for every verification across corners. Setting it toalways
saves them for every result that is stored in the database. Setting it tonever
disables saving pickled result files.parentId is the sqlite record id of the parent. If
None
the parent is the root database entry.This is a callable object with no arguments. The return value is a tuple comprising a dictionary with the final values of the design parameters, the
Aggregator
object used for evaluating the final result across all corners, and the analysisCount dictionary.Objects of this type store the number of analyses performed during the last call to the object in the analysisCount member.