1.3. pyopus.simulator.spectre
— Cadence Spectre simulator support¶
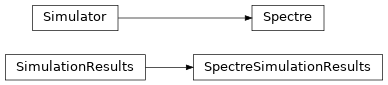
SPECTRE batch mode interface (PyOPUS subsystem name: SPSI)
SPECTRE is simulator from Cadence. It is capable of modifying circuit, subcircuit and simulator parameters, but cannot change the topology without restarting and loading a new file.
SPECTRE is not capable of changing the circuit’s topology (system definition) without restarting the simulator and loading a new input file. It also cannot have a different set of save directives for every analysis.
The temperature
parameter represents the circuit’s temperature in
degrees centigrade (options temp=...
simulator directive). Consequently
the temp
simulator option is not allowed to appear in the simulator
options list.
Save statements are global in SPECTRE. Therefore they apply to all analyses
in a file. If two analyses have different sets of save directives two separate
input files are generated when saveSplit is set to True
. Otherwise all
save directives from all jobs in a file are merged together.
Nutmeg rawfile (binary) files are used for collecting the reults.
A job sequence in SPECTRE is a list of lists containing the indices of jobs belonging to individual job groups.
One result group always contains only one plot. See
pyopus.simulator.rawfile
module for the details on the result files.
-
class
pyopus.simulator.spectre.
Spectre
(binary=None, args=[], debug=0, saveSplit=False)[source]¶ A class for interfacing with the SPECTRE simulator.
binary is the path to the SPECTRE simulator binary. If it is not given the
SPECTRE_BINARY
environmental variable is used as the path to the binary. IfSPECTRE_BINARY
is not set the binary is assumed to be named ‘spectre’ and located in the system PATH.args apecifies a list of additional arguments passed to the simulator binary at startup.
If debug is greater than 0 debug messages are printed at the standard output. If it is above 1 a part of the simulator output is also printed. If debug is above 2 full simulator output is printed.
The save directives from the simulator job description are evaluated in an environment where the following objects are available:
all
- a reference to thesave_all()
functionv
- a reference to thesave_voltage()
functioni
- a reference to thesave_current()
functionp
- a reference to thesave_property()
functionipath
- a reference to theipath()
function
Similarly the environment for evaluating the analysis command given in the job description consists of the following objects:
op
- a reference to thean_op()
functiondc
- a reference to thean_dc()
functionac
- a reference to thean_ac()
functiontran
- a reference to thean_tran()
functionnoise
- a reference to thean_noise()
functionipath
- a reference to theipath()
functionparam
- a dictionary containing the members of theparams
entry in the simulator job description together with the parameters from the dictionary passed at the last call to thesetInputParameters()
method. The parameters values given in the job description take precedence over the values passed to thesetInputParameters()
method.
Seting saveSplit to
True
splits a job group in multiple job groups with differing sets of save directives. Setting it toFalse
(default) joins the save directives from all jobs in a job group.-
cleanupResults
(i)[source]¶ Removes all result files that were produced during the simulation of the i-th job group. Simulator input files are left untouched.
-
classmethod
findSimulator
()[source]¶ Finds the simulator. Location is defined by the SPECTRE environmental variable. If the binary is not found there the system path is used.
-
optimizedJobSequence
()[source]¶ Returns the optimized job sequence.
Jobs in a job group have:
identical circuit definition,
identical save directives
-
readResults
(jobIndex, runOK=None)[source]¶ Read results of a job with given jobIndex.
runOK specifies the status returned by the
runJobGroup()
method which produced the results. If not specified the run status stored by the simulator is used.Returns an object of the class
SpiceOpusSimulationResults
. If the run failed or the results file cannot be read theNone
is returned.
-
runFile
(fileName)[source]¶ Runs the simulator on the input file given by fileName.
Returns
True
if the simulation finished successfully. This does not mean that any results were produced. It only means that the return code from the simuator was 0 (OK).
-
runJobGroup
(i)[source]¶ Runs the i-th job group.
First calls the
writeFile()
method followed by thecleanupResults()
method that removes any old results produced by previous runs of the jobs in i-th job group. Finally therunFile()
method is invoked. Its return value is stored in thelastRunStatus
member.The function returns a tuple (jobIndices, status) where jobIndices is a list of job indices corresponding to the i-th job group. status is the status returned by the
runFile()
method.
-
unoptimizedJobSequence
()[source]¶ Returns the unoptimized job sequence. If there are n jobs in the job list the following list of lists is returned:
[[0], [1], ..., [n-1]]
. This means we have n job groups with one job per job group.
-
writeFile
(i)[source]¶ Prepares the simulator input file for running the i-th job group.
The file is named
simulatorID_group_i.scs
where i is the index of the job group.All output files with simulation results are .raw files in binary format.
System description modules are converted to
include
simulator directives.Simulator options are set with the
set
simulator directive. Integer, real, and string simulator options are converted with the__str__()
method before they are written to the file. Boolean options are converted to0
or1t
depending on whether they areTrue
orFalse
.The parameters set with the last call to
setInputParameters()
method are joined with the parameters in the job description. The values from the job description take precedence over the values specified with thesetInputParameters()
method. All parameters are written to the input file in form ofalter
simulator directives.The
temperature
parameter is treated differently. It is written to the input file in form if aset
simulator directive preceding its corresponding analysis directive.Save directives are written as a series of
save
simulator directives.Every analysis command is evaluated in its corresponding environment taking into account the parameter values passed to the
setInputParameters()
method.All analyses write the results to a single binary .raw file.
The function returns the name of the simulator input file it generated.
-
class
pyopus.simulator.spectre.
SpectreSimulationResults
(rawData, params={}, variables={}, results={})[source]¶ Objects of this class hold Spectre simulation results.
-
driverTable
()[source]¶ Returns a dictionary of available driver functions for accessing simulation results.
-
ns
(reference, name=None, contrib=None)[source]¶ Retrieves the noise spectrum density of contribution contrib of instance name to the input/output noise spectrum density. reference can be
'input'
or'output'
.If name and contrib are not given the output or the equivalent input noise spectrum density is returned (depending on the value of reference).
In the simulator output file partial noise spectra are stored as squared noise (V^2/Hz or A^2/Hz). Total noise spectra are stored as V/sqrt(Hz) or A/sqrt(Hz). This function always returns squared noise with V^2/Hz or A^2/Hz as unit.
The spectrum is obtained from the resIndex-th plot.
-
p
(name, parameter, index=None)[source]¶ Retrieves the index-th component of property named parameter belonging to instance named name. index must always be
None
because Spectre does not support vector properties.Note that this works only of the property was saved with a corresponding save directive.
-
scale
(vecName=None)[source]¶ If vecName is specified returns the scale corresponding to the specified vector. Usually this is the default scale.
If vecName is not specified the default scale is returned.
-
scaleName
(vecName=None)[source]¶ If vecName is specified returns the name of the scale vector corresponding to the specified vector. Usually this is the default scale.
If vecName is not specified returns the name of the vector holding the default scale.
-
-
pyopus.simulator.spectre.
an_ac
(start=None, stop=None, sweep=None, points=None, **kwargs)[source]¶ Generats the SPECTRE simulator command that invokes a small signal (AC) analysis. See the SPECTRE manual for details on arguments.
sweep can be one of
'lin'
- linear sweep with the number of points given by points'dec'
- logarithmic sweep with points per decade (scale range of 1..10) given by points
if start, stop, sweep, and points are given a common PyOPUS ac sweep is performed.
Passes any additional arguments to the ac simulator directive.
-
pyopus.simulator.spectre.
an_dc
(start=None, stop=None, sweep=None, points=None, name=None, parameter=None, index=None, **kwargs)[source]¶ Generates the SPECTRE simulator command that invokes an operating point sweep (DC) analysis. See the SPECTRE manual for details on arguments.
Generates the SPECTRE simulator directive that invokes the operating point sweep (DC) analysis. start and stop give the intial and the final value of the swept parameter.
sweep can be one of
'lin'
- linear sweep with the number of points given by points'dec'
- logarithmic sweep with points per decade (scale range of 1..10) given by points
name gives the name of the instance whose parameter is swept. Because SPECTRE knows no such thing as vector parameters, index should never be used.
If name is not given a sweep of a circuit parameter (defined with
.param
) is performed. The name of the parameter can be specified with the parameter argument. If parameter istemperature
a sweep of the circuit’s temperature is performed.If star, stop, sweep, points, and parameter are given, a common PyOPUS dc sweep is performed. Otherwise the parameters are passed to the dc simulator directive.
Passes any additional arguments to the dc simulator directive.
-
pyopus.simulator.spectre.
an_noise
(start=None, stop=None, sweep=None, points=None, input=None, outp=None, outn=None, ptsSum=1, **kwargs)[source]¶ Generats the SPECTRE simulator command that invokes a small signal noise analysis. See the SPECTRE manual for details on arguments.
If start, stop, sweep, and points are given a common PyOPUS noise analysis is performed.
The ptsSum argument is ignored.
Passes any additional arguments to the noise simulator directive.
-
pyopus.simulator.spectre.
an_op
(**kwargs)[source]¶ Generates the SPECTRE simulator command that invokes an operating point analysis.
Passes any additional arguments to the dc simulator directive.
-
pyopus.simulator.spectre.
an_tran
(step=None, stop=None, start=0.0, maxStep=None, uic=False, **kwargs)[source]¶ Generats the SPECTRE simulator command that invokes a transient analysis. See the SPECTRE manual for details on arguments.
If step and stop (optionally start, maxStep, and uic) are given a common PyOPUS transient analysis is performed. To force a spectre style analysis with only stop (and start), specify at least one SPECTRE-only transient analysis parameter default value (e.g. skipdc=no).
Passes any additional arguments to the tran simulator directive.
-
pyopus.simulator.spectre.
ipath
(input, outerHierarchy=None, innerHierarchy=None, objectType='inst')[source]¶ Constructs a hierarchical path for the instance with name given by input. The object is located within outerHierarchy (a list of instances with innermost instance listed first). innerHierarchy a list of names specifying the instance hierarchy inner to the input instance. The innermost instance name is listed first. If outerHierarchy is not given input is assumed to be the outermost element in the hierarchy. Similarly if innerHierarchy is not given input is assumed to be the innermost element in the hierarchy.
Returns a string representing a hierarchical path.
If input is a list the return value is also a list representing hierarchical paths corresponding to elements in input.
innerHierarchy and outerHierarchy can also be ordinary strings (equivalent to a list with only one string as a member).
The objectType argument is for compatibility with other simulators. Because SPICE OPUS treats the hierarchical paths of all objects in the same way, the return value does not depend on objectType. The available values of objectType are
'inst'
,'mod'
, and'node'
.SPICE OPUS hierarchical paths begin with the innermost instance followed by its enclosing instances. Colon (
:
) is used as the separator between instances in the hierarchy. Som1:x1:x2
is an instance namedm1
that is a part ofx1
(insidex1
) which in turn is a part ofx2
(insidex2
).Some examples:
ipath('m1', ['x1', 'x2'])
- instance namedm1
insidex1
insidex2
. Returns'x2.x1.m1'
.ipath('x1', innerHierarchy=['m0', 'x0'])
- instancem0
insidex0
insidex1
. Returns'x1.x0.m0'
.ipath(['m1', 'm2'], ['x1', 'x2']) - instances ``m1
andm2
insidex1
insidex2
. Returns['x2.x1.m1', 'x2.x1.m2']
.ipath(['xm1', 'xm2'], ['x1', 'x2'], 'm0')
- instances namedm0
inside pathsx2.x1.xm1
andx2.x1.xm2
. Returns['x2.x1.xm1.m0', 'x2.x1.xm2.m0']
.
-
pyopus.simulator.spectre.
save_all
()[source]¶ Returns a save directive that saves all results the simulator normally saves in its output (in SPICE OPUS these are all node voltages and all currents flowing through voltage sources and inductances).
-
pyopus.simulator.spectre.
save_current
(what, terminal=1)[source]¶ If what si a string it returns a save directive that instructs the simulator to save the current flowing through instance names *what in simulator output. If what is a list of strings multiple save diretives are returned instructing the simulator to save the currents flowing through instances with names given by the what list. terminal specifies the number of the terminal it which the current is saved.
-
pyopus.simulator.spectre.
save_property
(devices, params, indices=None)[source]¶ Saves the properties given by the list of property names (params) of instances given by the devices list.
If params and the devices have n and m members, n*m save directives are returned describing all combinations of device name and property name.
-
pyopus.simulator.spectre.
save_voltage
(what)[source]¶ If what is a string it returns a save directive that instructs the simulator to save the voltage of node named what in simulator output. If what is a list of strings a multiple save directives are returned instructing the simulator to save the voltages of nodes with names given by the what list.
Equivalent of SPICE OPUS
save v(what)
simulator command.