1.1. pyopus.simulator.base
— Base class for simulator objects¶
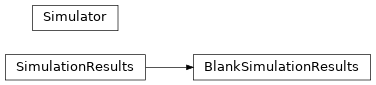
Base class for simulator objects (PyOPUS subsystem name: SI)
A system is a physical object (e.g. a circuit) whose characteristics and responses are evaluated (simulated) by a simulator.
Simulators are divided into two groups: batch mode simulators and interactive simulators. An interactive simulator presents the user with a prompt where commands of a simulator-specific command language can be entered to control the simulator (load input file, perform simulation, save result file, …).
Interactive simulators can be used as batch simulators if they are presented with a script written in the simulator control language at their standard input.
To tell a batch mode simulator what to simulate one must provide a job list. A job list is a list of jobs where every job specifies one simulation.
A job is a dictionary with the following members:
name
- the name of the jobdefinitions
- a list of system description modules that constitute the system to be simulatedparams
- a dictionary with the system parameters used for this job. Keys are parameter names while values are parameter valuesoptions
- a dictionary with the simulator options used for this job. Keys are option names while values are option valuesvariables
- a dictionary containing the Python variables that are available during the evaluation ofsaves
andcommand
.saves
- a list of strings giving Python expressions that evaluate to lists of save directives specifying what simulated quantities should be stored in simulator outputcommand
- a string giving a Python expression that evaluates to the simulator command for the analysis performed by this job
The order in which jobs are given may not be optimal for fastest simulation because it can require an excessive number of system parameter/system description changes. The process of job optimization reorders and groups the jobs into job groups in such manner that the number of these changes is minimized. the sequence of job groups is called optimized job sequence Optimal job ordering and grouping as well as the way individual groups of jobs are handled in simulation depends on the simulator.
A batch simulator offers the capability to run the jobs in one job group at a
time. For every job group the simulator is started, jobs simulated, and the
simulator shut down. Every time a job group is run the user can provide a
dictionary of inputParameters that complement the parameters defined in the
params
dictionaries of the jobs. If both the inputParameters and the
params
dictionary of a job list the value of the same parameter, the value
in the params
dictionary takes precedence over the value defined in the
inputParameters dictionary. After the simulation in finished the results can
be retrieved from the simulator object.
Interactive simulators offer the user the possibility to send commands to the simulator. After the execution of every command the output produced by the simulator is returned. Collecting simulator results is left over to the user. The simulator can be shut down and restarted whenever the user decides.
-
class
pyopus.simulator.base.
BlankSimulationResults
(params={}, variables={}, results={})[source]¶ Results object used for evaluating measures that are not associated with an analysis (i.e. dependent measures).
-
evalEnvironment
()[source]¶ Returns the environment in which measures will be evaluated. The environment consists of following entries:
Python variables defined for the simulation that produced the results.
m
-pyopus.performance.measure
modulenp
-numpy
moduleparam
- parameter values dictionary. These parameters were used during simulation that produced the resultsresult
- double dictionary with measure results. First key is measure, second key is corner.driver functions returned by the
driverTable()
method.
The environment is constructed in this order. Later entries override earlier entries if entry name is the same.
-
-
class
pyopus.simulator.base.
SimulationResults
(params={}, variables={}, results={})[source]¶ Base class for simulation results.
-
driverTable
()[source]¶ Returns a dictionary of available driver functions for accessing simulation results.
They are used for measurement evaluation in
PerformanceEvaluator
objects where they appear as a part of the environment for evaluating the measures.The following functions (dictionary key names) are usually available:
ipath
- a reference to theipath()
function for constructing object paths in hierarchical designsvectorNames
- returns the list of available raw vectorsvector
- retrieve a vector by its raw namev
- retrieve a node potential or potential difference between two nodesi
- retrieve a current flowing through an instance (usually a voltage source or inductor)p
- retrieve an element instance property (assuming that it was saves during simulation with a correspondign save directive)ns
- retrieve a noise spectrumscaleName
- retrieve the name of the vector holding the scale corresponding to a vector or the default scale of a results groupscale
- retrieve the scale corresponding to a vector or the default scale of a results group
-
evalEnvironment
()[source]¶ Returns the environment in which measures will be evaluated. The environment consists of following entries:
Python variables defined for the simulation that produced the results.
m
-pyopus.performance.measure
modulenp
-numpy
moduleparam
- parameter values dictionary. These parameters were used during simulation that produced the resultsdriver functions returned by the
driverTable()
method.
The environment is constructed in this order. Later entries override earlier entries if entry name is the same.
-
param
(name=None)[source]¶ Returns a stored PyOPUS parameter with given name.
If name is not specified returns the dictionary of all stored parameters.
-
result
(measure=None, corner=None)[source]¶ Returns a stored PyOPUS measure value named name for given corner.
If only measure is specified returns a dictionary holding values of that measure across all corners. If measure does not exist and error is raised.
If only corner is specified returns a dictionary holding all measures for that particular corner. If no such corner exists in the results dictionary an error is raised.
if neither measure nor corner is specified, returns the dictionary holding all stored measure values across all corners with first index being the measure name and the second one the corner name.
-
-
class
pyopus.simulator.base.
Simulator
(binary, args=[], debug=0)[source]¶ Base class for simulator classes.
Every simulator is equipped with a simulatorID unique for every simulator in every Python process on every host. The format of the simulatorID is
<locationID>_simulatorObjectNumber
<locationID>
is generated by thepyopus.misc.locationID()
function. It containes the information on the hosts and the task within which the simulator object was created.A different simulator object number is assigned to every simulator object of a given type in one Python interpreter.
All intermediate files resulting from the actions performed by a simulator object have their name prefixed by simulatorID.
Every simulator can hold result groups from several jobs. One of these groups is the active result group.
The binary argument gives the full path to the simulator executable. args is a list of additional command line parameters passed to the simulator executable at simulator startup.
If debug is greater than 0 debug messages are printed to standard output.
-
cleanup
()[source]¶ Removes all intermediate files created as a result of actions performed by this simulator object (files with filenames that have simulatorID for prefix).
-
cleanupResults
(i)[source]¶ Removes all result files that were produced during the simulation of the i-th job group. Simulator input files are left untouched.
-
optimizedJobSequence
()[source]¶ Returns the optimized job sequence. Usually the order of job groups and jobs within them is identical to the order of jobs in the job list set with the
setJobList()
method.
-
readResults
(jobIndex, runOK=None)[source]¶ Read results of job with given jobIndex.
runOK specifies the status returned by the
runJobGroup()
method which produced the results.
-
runJobGroup
(i)[source]¶ Runs the jobs in the i-th job group. Returns a tuple of the form (jobIndices, status) where jobIndices is a list of indices corresponding to the jobs that ran. status is
True
if everything is OK.
-
setInputParameters
(inParams)[source]¶ Sets the dictionary of input parameters to inParams. These parameters are used in all subsequent batch simulations.
-
setJobList
(jobList, optimize=True)[source]¶ Sets jobList to be the job list for batch simulation. If the
options
,params
,saves
, orvariables
member is missing in any of the jobs, an empty dictionary/list is added to that job.The job list is marked as fresh meaning that a new set of simulator input files needs to be created. Files are created the first time a job group is run.
If optimize is
True
the optimized job sequence is computed by calling theoptimizedJobSequence()
method. If optimize isTrue
an unoptimized job sequence is produced by calling theunoptimizedJobSequence()
method.
-
stopSimulator
()[source]¶ Stops a running interactive simulator. This is done by politely asking the simulator to exit.
-
unoptimizedJobSequence
()[source]¶ Returns the unoptimized job sequence. A job sequence is a list of lists containing indices in the job list passed to the
setJobList()
method. Every inner list corresponds to one job group.
-